Recently while writing JavaScript (not jQuery) code, I required the use of a set. In Java, this functionality is provided by the Set collection.
The specifics of which are a collection of object where no duplicates are allowed. As no such object exists (to my knowledge) in JavaScript I wrote it myself *smug*.
To implement a set in JavaScript I created an array. I do not want any duplicates in this array and I want to be able to check if an item already exists in the array.
Lets say I have a list of cars.
var car_1 = ‘Ford’;
var car_2 = ‘Nissan’;
var car_3 = ‘Mitsubishi’;
var car_4 = ‘Nissan’;
I want to create an array of cars but with no duplicates:
var cars = []; //an empty array
If I want to add a car to the set (array named cars), only if it does not already exist in the JavaScript set I do so like so:
cars = growSet(cars,car_1);
The growSet function:
/*takes a setname and an item to add to said set. Returns the set with the item added to it.no duplicates */
function growSet(setName,setUnit){
var exists = false;
for(var i = 0; i<setName.length; i++){
if((setName[i]==setUnit)){
exists = true;
}
}
if(exists==false){
if(!(setUnit==’Select All’))
setName.push(setUnit);
}
return setName;
}
If I want to check if a car exists in the set (array named cars), I do so like so:
existsInSet(cars,car_1);
The boolean returning existsInSet function:
/*checks weather or not an item exists in a set without adding/removing said item to/from the set */
function existsInSet(setName,setUnit){
var exists = false;
for(var i = 0; i<setName.length; i++){
if((setName[i]==setUnit)){
exists = true;
}
}
return exists;
}
If I want to remove a car from the set (array named cars), I do so like so:
cars = shrinkSet(cars,car_1);
The shrinkSet function:
/*takes a setname and an item to remove from said set. Returns the set with the item removed from it. */
function shrinkSet(setName,setUnit){
var newSet = [];
for(var i = 0; i<setName.length; i++){
if(setName[i]==setUnit){
//do nothing
}else{
newSet.push(setName[i]);
}
}
return newSet;
}
Finally, enjoy a picture of Dennis Bergkamp.
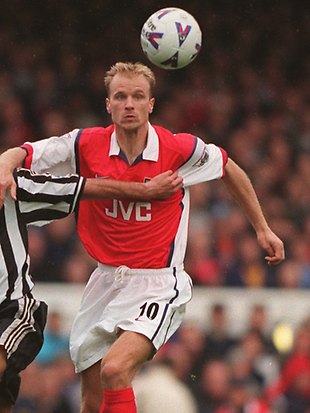
Dennis Bergkamp, would rather fly than have duplicates in his JavaScript arrays.